- Invade the Code π½
- Posts
- π Day 19 of #100DaysOfCode: Conquering Integration Testing with Testcontainers! ππ³
π Day 19 of #100DaysOfCode: Conquering Integration Testing with Testcontainers! ππ³
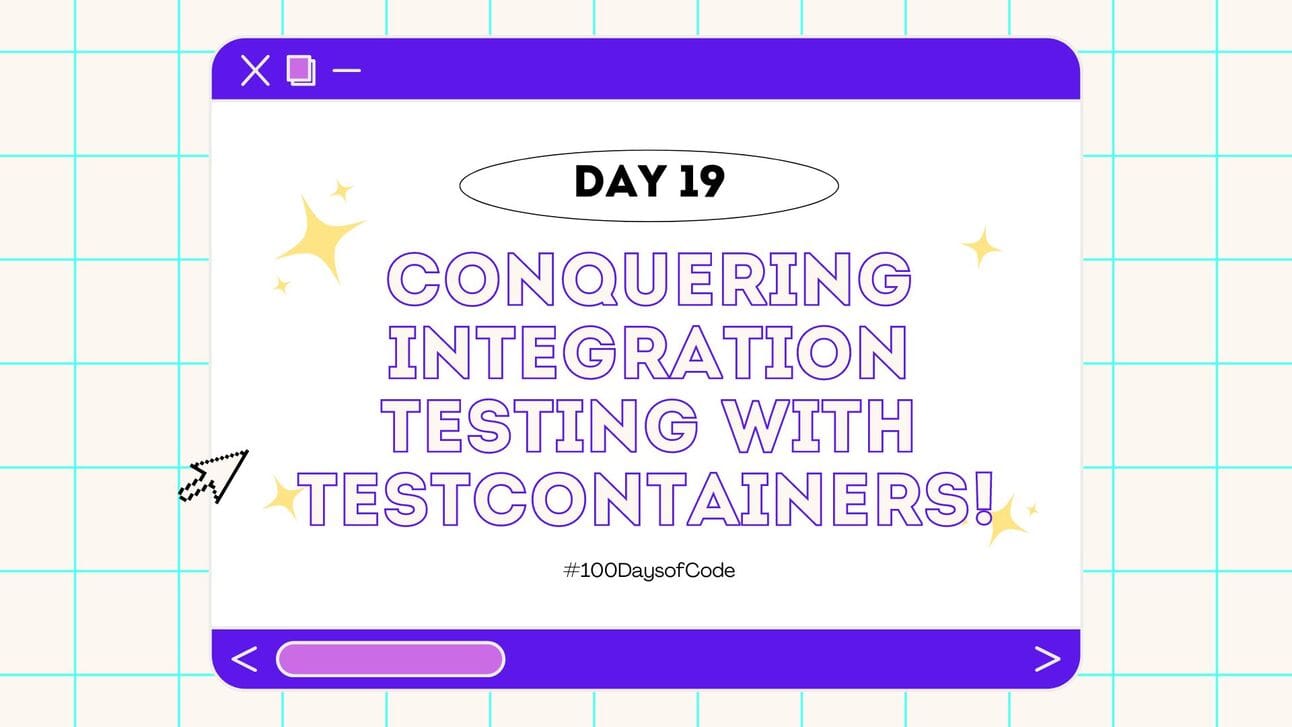
Hey Invaders! π½
#100daysofcode day 19: Today marks a milestone as I wrap up the JUnit course with a deep dive into Integration Testing using Testcontainers. This Java library revolutionizes testing by leveraging Docker containers to simulate external services like databases, allowing for highly realistic tests. π
Despite a persistent issue with the @ServiceContainer
annotation that stumped me for now ("Cannot resolve symbol 'ServiceContainer'"), I gained valuable insights into setting up and managing Docker containers for testing environments. Hereβs a peek at how I intended to structure the tests:
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@Testcontainers
@TestMethodOrder(MethodOrderer.OrderAnnotation.class)
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class UsersControllerWithTestcontainersTest {
@Autowired
private TestRestTemplate testRestTemplate;
private String authorizationToken;
@ServiceContainer
private static MySQLContainer mySQLContainer = new MySQLContainer("mysql:8.4.0").withExposedPorts(3306);
static {
mySQLContainer.start();
}
@Order(1)
@Test
@DisplayName("The MySQL container is created and is running")
void testContainerIsRunning() {
assertTrue(mySQLContainer.isCreated(), "MySQL container should be created");
assertTrue(mySQLContainer.isRunning(), "MySQL container should be running");
}
@Order(2)
@Test
@DisplayName("User can be created")
void testCreateUser_whenValidDetailsProvided_returnsUserDetails() throws JSONException {
// Arrange
JSONObject userDetailsRequestJson = new JSONObject();
userDetailsRequestJson.put("firstName", "Sergey");
userDetailsRequestJson.put("lastName", "Kargopolov");
userDetailsRequestJson.put("email", "[email protected]");
userDetailsRequestJson.put("password","12345678");
userDetailsRequestJson.put("repeatPassword", "12345678");
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
headers.setAccept(Arrays.asList(MediaType.APPLICATION_JSON));
HttpEntity<String> request = new HttpEntity<>(userDetailsRequestJson.toString(), headers);
// Act
ResponseEntity<UserRest> createdUserDetailsEntity = testRestTemplate.postForEntity("/users",
request,
UserRest.class);
UserRest createdUserDetails = createdUserDetailsEntity.getBody();
// Assert
assertEquals(HttpStatus.OK, createdUserDetailsEntity.getStatusCode(), "HTTP status should be 200 OK");
assertEquals(userDetailsRequestJson.getString("firstName"), createdUserDetails.getFirstName(), "First name should match input");
assertEquals(userDetailsRequestJson.getString("lastName"), createdUserDetails.getLastName(), "Last name should match input");
assertEquals(userDetailsRequestJson.getString("email"), createdUserDetails.getEmail(), "Email should match input");
assertFalse(createdUserDetails.getUserId().trim().isEmpty(), "User ID should not be empty");
}
}
Even though I faced challenges with version compatibility or annotation mishaps, the hands-on experience has been invaluable. I'll revisit the problematic areas once I update my dependencies or consult further documentation. For now, Iβm excited to apply these robust testing practices to future projects, ensuring high-quality software delivery. ππ οΈ
You can further look into my files on Git Hub for this course and maybe you can find the culprit of why @ServiceContainer isnβt working for me or what annotation I should be using instead!
Reply